LLM(Learning Language Models) has made a big entry in recent times. They have applications in a wide range of domains. One of which is web development.
GPT-3, one of the popular LLMs, can be used to tackle some of the day-to-day challenges for web developers. Given the fact web developers spend considerable time searching the web, this can cut down the time to handle tasks.
Let me cover a few in this article. I have given GPT-3 specific instructions for common development tasks. You can use these on the OpenAI playground or directly on your Mac with the Elephas app.
Debugging an error
So you run your code and get a weird error that does not make sense at first. You can ask GPT-3 to answer to it. Simply giving the prompt,
Explain in detail why this error shows up and ways to fix it.
Here is an error in a python code to test it,

I got,
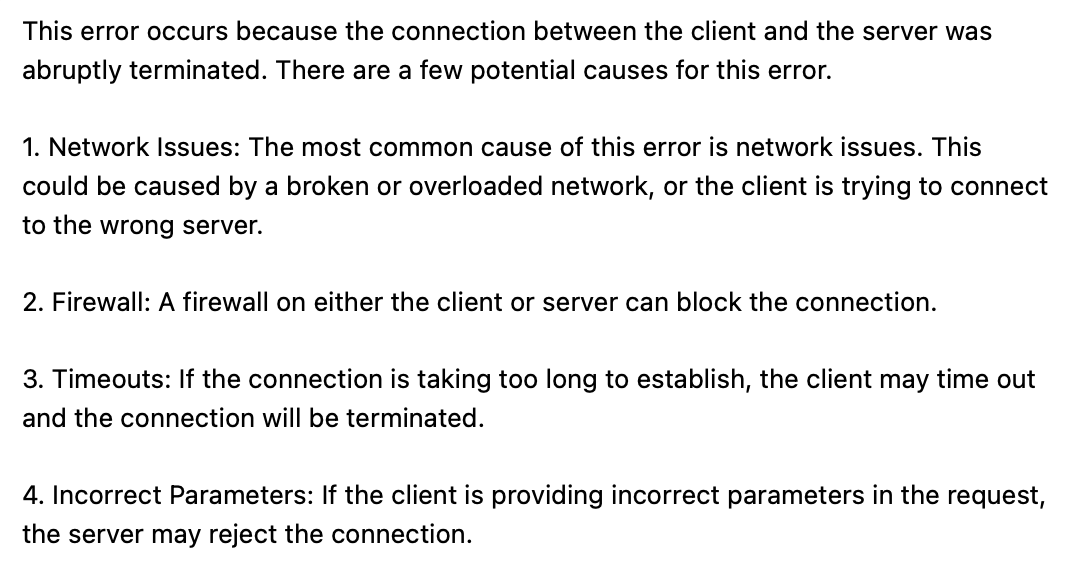
Generating SQL
When you need to write SQL and have clear instructions, you can easily turn into a SQL query with GPT-3.
Turn the instruction to a MySQL query.
You can replace with the respective database type, such as, Postgres or SQL Server.
Let's say I want to generate a MySQL query for this requirement,
Fetch the three highest salaries from the Worker table.
When you run the instruction above,
SELECT salary FROM Worker ORDER BY salary DESC LIMIT 3;
Note: I use Elephas.app's snippet feature to run GPT-3 everywhere on my Mac.
Translate code
Want to translate code from one programming language to another? That would normally require hours for even a simple program. Now, GPT-3 can translate them in seconds. Here is an instruction you can use,
Translate the following code Python.
Here is a sample PHP code I gave,
<?php$filename = 'cars.csv';// The nested array to hold all the arrays$the_big_array = [];// Open the file for readingif (($h = fopen("{$filename}", "r")) !== FALSE){// Each line in the file is converted into an individual array that we call $data// The items of the array are comma separatedwhile (($data = fgetcsv($h, 1000, ",")) !== FALSE){// Each individual array is being pushed into the nested array$the_big_array[] = $data;}// Close the filefclose($h);}
and the output I got,
import csv
filename = 'cars.csv'
# The nested list to hold all the lists
the_big_list = []
# Open the file for reading
with open(filename, 'r') as f:
# Each line in the file is converted into an individual list that we call data
# The items of the list are comma separated
data = csv.reader(f, delimiter=',')
# Each individual list is being pushed into the nested list
for row in data:the_big_list.append(row)
# Display the code in a readable format
print(the_big_list)
In a few cases, you may need to tweak a bit.
Enforcing coding standards
When you want to make sure your code follows the coding standards, you can use GPT-3 to update it as required.
Instruction,
Convert this PHP code to PSR-4 standard but without changing the logic or used methods.
Input code,
<?php
$num = mt_rand(100000,999999);
printf("%d", $num);
?>
The output I got,
namespace App;
class RandomNumber
{
public function generate()
{
$num = mt_rand(100000, 999999);
printf("%d", $num);
}
}
Boilerplate code
You don't want to write all the basic code. What if you can generate boilerplate code easily for common tasks?
Write a bootstrap 3 boilerplate code for a website. 5 menus, three body region, and a footer.
Output included the full HTML. I would double the external links before using the code.
Understanding code
You can understand any code with GPT-3. If you use Elephas, you can get explanation for code across apps. Here is the instruction you can use,
Explain the following code as if I were a new developer.
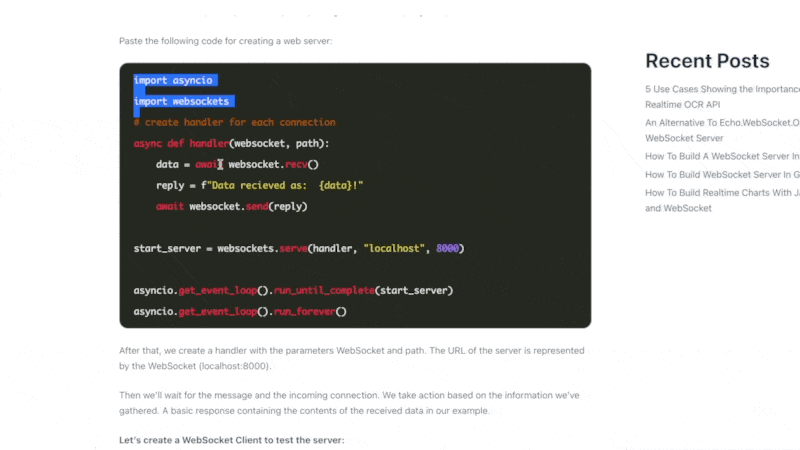
Slow code?
Find bottleneck in your code with ease.
Explain why the following code is slow and propose better ways.
Input code,
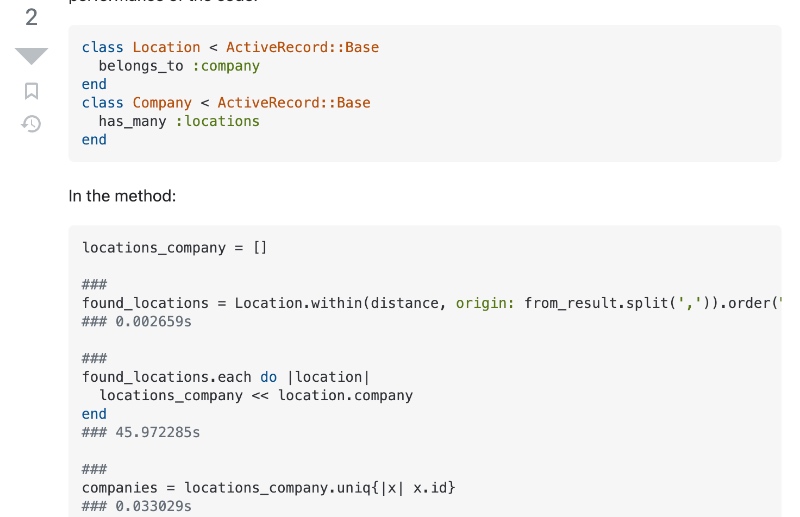
Recommendations,
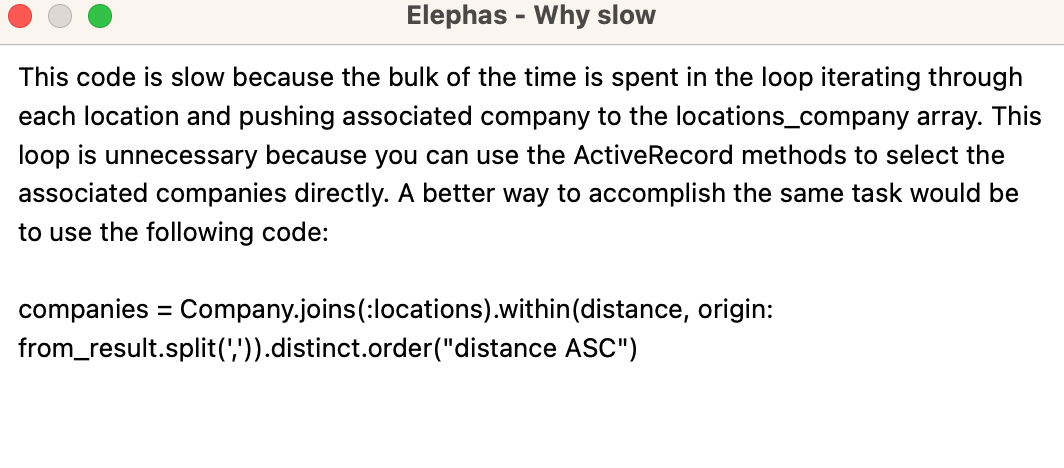
Write documentation
You can have GPT-3 write technical use cases.
We wish to develop a broad-ranging corpus of regular expressions that tests a variety of real-world use cases. Give 10 general categories that would be appropriate for organizing this corpus and several subcategories for each. Give your response as a hierarchically numbered list.
This might produce,
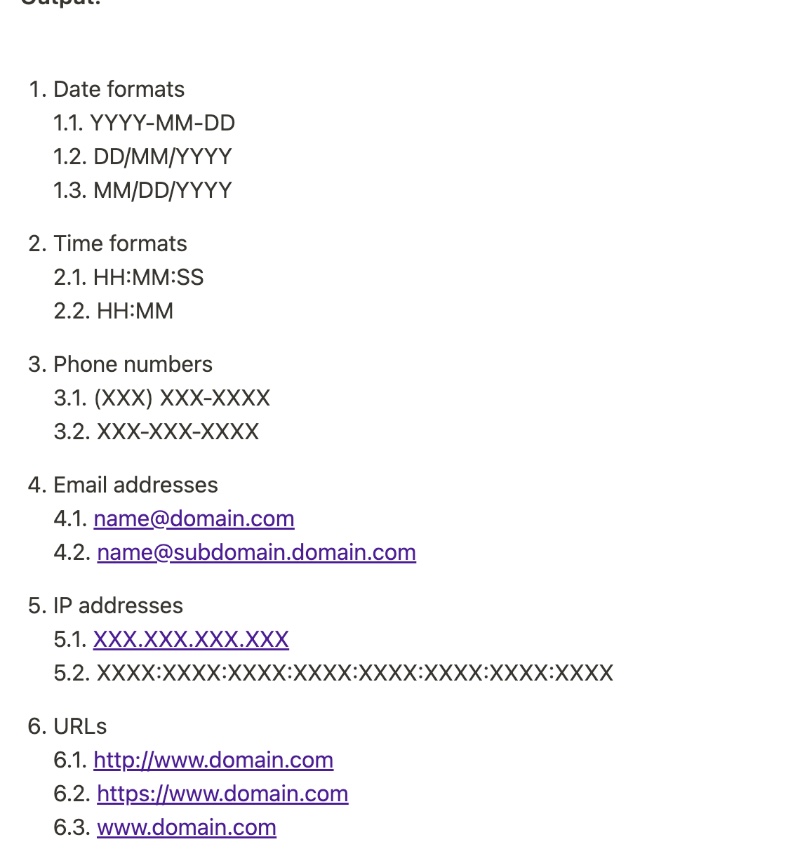
I hope you find this useful. Check out https://elephas.app, which lets you use GPT-3 as a layer on top of your Mac to save you hours every day.